How to show the Status of a Binding
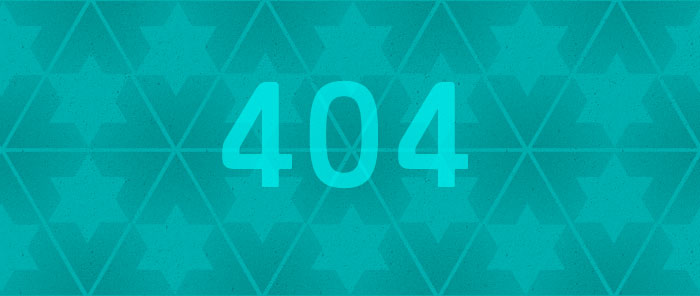
Sometimes it takes a while to retrieve the source data of a Binding. This is especially true if your application relies on data from a web service or rss feed on the web. In those situations, you will probably want to show information about the status of the binding to the user: Are you still waiting for the data to load? Was there an error when loading it? The Data Binding team has been discussing the possibility of adding a Status property on DataSourceProvider for V2, but until then, we have to come up with our own custom solutions. Today I will show you one way of displaying the status of a Binding in an application.
This application will start by binding to an invalid URL that returns a 404 error. I will show you how to get and display the exception error message when things don’t go as expected. I will then replace the source URL with a valid one, causing all the bindings to show the information I’m looking for. There are 3 status messages in this application: “Opening feed…”, “Ready” and the message of the exception. Here is the order in which I expect them to come up:
“Opening feed…” -> (404 error) -> “The remote server returned an error: (404) Not Found.” -> (Click button to fix source URL) -> “Opening feed…” -> (Valid data is loaded) -> “Ready” -> (Click Refresh button) -> “Opening feed…” -> (Valid data is loaded) -> “Ready”
The correct Uri that causes all bindings to succeed is http://xml.weather.yahoo.com/forecastrss?p=98052, which contains information about the weather conditions in Redmond. If you look at the xml in this source carefully, you will notice that several elements belong to one of the namespaces specified at the top of the file, as you can see below for the “condition” element:
<rss version="2.0" xmlns:yweather="http://xml.weather.yahoo.com/ns/rss/1.0" xmlns:geo="http://www.w3.org/2003/01/geo/wgs84_pos#">
(…)
<yweather:condition text="Fair" code="34" temp="62" date="Sun, 23 Apr 2006 11:53 am PDT" />
How can we bind to the condition’s text in Avalon? We can do this by setting the XmlNamespaceManager property of XmlDataProvider to an XmlNamespacesMappingCollection, which contains one or more XmlNamespaceMappings. The mappings’ Uri has to map to the Uri in the source xml data, but the Prefix can be whatever you want (although I usually get the prefixes to map too to avoid confusion). We want to be independent of the source’s prefixes so that your Avalon application won’t break if the owners of the source decide to change their prefixes.
<Window.Resources>
<XmlNamespaceMappingCollection x:Key="namespace">
<XmlNamespaceMapping Prefix="yweather" Uri="http://xml.weather.yahoo.com/ns/rss/1.0" />
</XmlNamespaceMappingCollection>
<XmlDataProvider (…) XmlNamespaceManager="{StaticResource namespace}" />
(…)
</Window.Resources>
Once you have the xml mappings in place, binding to an element within a namespace is as simple as specifying the namespace before the name of the element:
<Label Grid.Row="2" Grid.Column="1" Content="{Binding XPath=item/yweather:condition/@text}" HorizontalAlignment="Left"/>
I want to display the correct status to the user in the StatusBar of the Window. I added a string property to the Window called “StatusMessage”, which will contain the strings “Opening feed…”, “Ready” or, in case of error, the error message of the exception. I then bound the StatusBar to this property as you can see below:
<StatusBar DockPanel.Dock="Bottom">
<StatusBarItem Name="statusBar">
<StatusBarItem.Content>
<Binding ElementName="win" Path="StatusMessage" />
</StatusBarItem.Content>
</StatusBarItem>
</StatusBar>
The next step is to set the StatusMessage property to the correct status string. I started by defined two constants with the status messages that will be displayed when the page is opening and ready:
private const string openingMessage = "Opening feed…";
private const string readyMessage = "Ready";
Then I started thinking about which actions taken by the user cause the application to be in a particular state. If this becomes confusing for your application, I recommend drawing a state machine by identifying all states, actions and interaction between the two. I use this approach frequently when writing automation tests for data binding scenarios, especially when they’re complex. What actions cause this app to be in the opening state? Beginning the application, clicking the button to fix the URL and clicking the button to Refresh:
public Window1()
{
this.StatusMessage = openingMessage;
InitializeComponent();
}
void FixSource(object sender, RoutedEventArgs args)
{
xdp.Source = new Uri("http://xml.weather.yahoo.com/forecastrss?p=98052");
this.StatusMessage = openingMessage;
}
void Refresh(object sender, RoutedEventArgs args)
{
xdp.Refresh();
this.StatusMessage = openingMessage;
}
What causes the application to be in the ready state? Getting new data. There is a DataChanged event handler in XmlDataProvider that can be used when we want to be alerted that new data was found:
protected override void OnInitialized(EventArgs e)
{
base.OnInitialized(e);
xdp = (XmlDataProvider)(this.Resources["404Provider"]);
(…)
xdp.DataChanged += DataChanged;
}
void DataChanged(object sender, EventArgs e)
{
this.StatusMessage = readyMessage;
}
There is no event in XmlDataProvider specifically for informing us that the Error property changed. However, XmlDataProvider implements INotifyPropertyChanged and the PropertyChanged event is raised when Error changes. As a side note, keep in mind that a class implementing INotifyPropertyChanged is not a promise that it will raise PropertChanged when every single one of its properties changes: a class could have 10 properties, and only raise PropertyChanged for one of them. But in this case this is not a problem, XmlDataProvider does raise a PropertyChanged when Error changes. We can then provide a handler for PropertyChanged and set the StatusMessage to the error message when an exception is set to the Error property:
protected override void OnInitialized(EventArgs e)
{
(…)
((INotifyPropertyChanged)xdp).PropertyChanged += SourceChanged;
(…)
}
void SourceChanged(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "Error")
{
if (xdp.Error != null)
{
(…)
this.StatusMessage = xdp.Error.Message;
}
(…)
}
}
I used the PropertyChanged handler to control the visibility of the Refresh and Fix buttons. This way, they only appear when it makes sense to click on them:
void SourceChanged(object sender, PropertyChangedEventArgs e)
{
if (e.PropertyName == "Error")
{
if (xdp.Error != null)
{
refreshButton.Visibility = Visibility.Collapsed;
fixButton.Visibility = Visibility.Visible;
this.StatusMessage = xdp.Error.Message;
}
else
{
fixButton.Visibility = Visibility.Collapsed;
refreshButton.Visibility = Visibility.Visible;
}
}
}
And last, I used the setter of StatusMessage to display the hourglass cursor when the application is in the opening state:
private string statusMessage;
public string StatusMessage
{
get { return statusMessage; }
set
{
statusMessage = value;
if (statusMessage == openingMessage)
{
this.Cursor = Cursors.Wait;
}
else
{
this.Cursor = null;
}
OnPropertyChanged("StatusMessage");
}
}
Here are a few screenshots of different states of this application:
Here you can find the VS project with this sample code. This works with February CTP WPF bits.
tom greasley
Is there a way to monitor the progress of the binding action itself?
For example, I have a large, deeply nested XML file that i’m binding to a treeview using hierarchical data templates.
Some of the nodes have many children (200 or so) and when they are expanded for the first time the app freezes whilst the binding is taking place and, I assume, the nodes are being created and drawn. This can take some time.
I’d like to, at least, be able to display an hourglass whilst this is happing but can’t find any suitable events to use.
April 24, 2006 at 7:18 am
Bea
There is an event in ItemContainerGenerator - StatusChanged - that tells you when it has finished generating the items for a particular container. When the Status property changes to “ContainersGenerated” you know you can stop your hourglass. You can get the ItemContainerGenerator for an ItemsControl through its ItemContainerGenerator property.
Although we provide the information you need, this is not a straight-forward problem to implement for TreeView. The problem is that TreeView has many generators: the TreeView itself plus each TreeViewItem derive from ItemsControl and contain their own generator.
I’d be interested in knowing if you are able to successfully implement a solution for this. I may give it a try if I have some free time.
April 25, 2006 at 9:19 am
tom greasley
Many thanks. That is a very useful hint at where to start. I’ll let you know about anything I come up with.
Ultimately it would be nice to have the actual binding itself done asynchronously so the UI does not block. Is this something we (the general public) can do with enough knowledge of WPF data binding?
April 26, 2006 at 8:06 am
Bea
Hi Tom,
Replying to your last question evolved into my last blog post. Thanks for the idea
I hope you find it useful.
Bea
May 21, 2006 at 1:51 pm
Keith
Out of curiosity, what ever happened re: the discussions within the DSP team about the Status property? I’m staring at a 3.5 app with the same problem (although I’d prefer routed events to a a status property for localization reasons)
July 1, 2009 at 2:35 pm
Bea
Hi Keith,
Unfortunately, the Status property was never added… The solution in this blog post should still be useful with current bits.
Bea
November 20, 2009 at 1:56 pm